[ You are viewing an archive of the CozyNet blog. ]
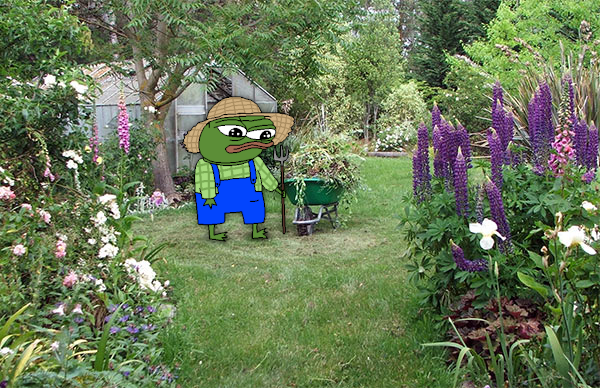
Welcome to the CozyNet Blog!
Muh blog post PHP navigator script
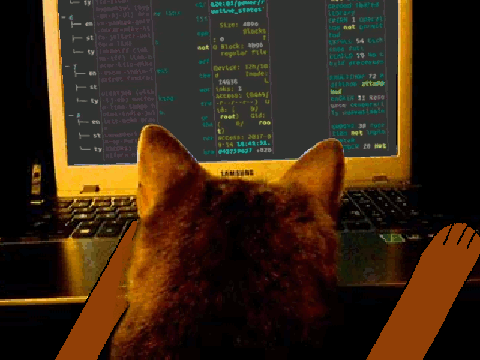
Literally MeOwww
As I've said before, I’m just learning shid as I go here. I have an idea of what I want based off past site examples, but I’m also limited by what I know and what I can do with what I know. I’ve managed to come up with a few useful scripts after reading various stack exchange threads. After some tweaking and a little re-working, they’ve proven to be useful, so I'll share a few here and explain how they work!
The PHP script
The following script will produce two buttons, just like these:
Now the method I’m going to present here is probably not the best. I believe that containing a list of file names within a table on a database and pulling from that would be much cleaner, but dealing with databases is a lesson in and of itself; so, I’m going to be a little unorthodoxed here and suggest using index files instead!
In case you’re wondering what an “index” file is, it’s basically what I'm referring to as a text file with a list of stuff in it (I'm NOT talking about an index.html web doc.) I call it an “index” file because that’s its purpose, to serve as an index. I don't know if there's a better name for it...
Over time, this would have to be converted into pulling from a database, but if your blog remains small, then it’s not so bad to keep an index with a few hundred lines of text. Keep in mind that for every site visitor, this index file will have to be read by the script; if your index file grows into a few megabytes, then consider what might could happen if you had a few thousand visitors hit your site all at once. You would quickly reach the limit of your memory and it would hose up!
Anyhow, enough about index files. Here's the PHP script.
<?php
$page = $src_name;
$files = file('/var/www/www.cozynet.org/db/blog_index.db', FILE_IGNORE_NEW_LINES);
$currentIdx = array_search($page, $files);
$nextIdx = $currentIdx + 1;
$prevIdx = $currentIdx - 1;
if (isset($files[$nextIdx])) {
$nextFile = $files[$nextIdx];
$next = pathinfo($nextFile, PATHINFO_BASENAME);
echo '<a class="sitenav_button" href="'.$next.'">'."«".'</a>'; }
else {
echo '<a class="sitenav_button_disabled">«</a>'; }
if (isset($files[$prevIdx])) {
$prevFile = $files[$prevIdx];
$prev = pathinfo($prevFile, PATHINFO_BASENAME);
echo '<a class="sitenav_button" href="'.$prev.'">'."»".'</a>'; }
else {
echo '<a class="sitenav_button_disabled">»</a>'; }
?>
How it works:
- The $page = $src_name variable is unnecessarily redundant, but I did that for the sake of comprehensibility later in the script. In case you're wondering, the $src_name variable is set outside of the above PHP script, which I'll get to in the next part that demonstrates the HTML side. This is an important part so that the script will understand which document you’re coming from, otherwise how will it know which is the next or previous page? I don’t recommend using session cookies for that, it’s a shitty practice to tie functionality like this into a cookie. Better to do it this way!
- The $files variable is using the PHP “file” function to read from the “blog_index.db” file, storing its contents into an array. The highlighted red file path will need to be adjusted to your file path. The “FILE_IGNORE_NEW_LINES” is necessary, or else the next function “array_search” will shit itself because it hates new lines.
- The $currentIdx variable is using the array_search function to locate $page within the $files array. Array items are assigned to numbers, so it’s searching for where in the array $page exists.
- The $nextIdx and $prevIdx variables are using basic math to determine the next and previous array items based off the result of $currentIdx. So if you’re presently viewing blog post 9 out of 20 blog posts total, then 8 is the previous and 10 is the next. Simple!
- Then the script does a check to see if the variables for $nextIdx and $prevIdx are set, else create a button with a disabled effect using CSS classes. This part is up to you and however you choose to theme your buttons. If you’re wondering what would happen if you reach the end of either side of the array (list of blog posts), the $nextIdx or $prevIdx will be empty and the if condition will default to else.
HTML stuff
The following snippet is an example of how to include the above PHP script into an HTML web document. The ideal is that you would store the whole script outside of the webroot, then call it up from within the specified web document, but it’s up to you however you want to approach it. I recommend this method so that you can apply changes to the script in the future without having to modify hundreds of HTML documents individually.
<html>
<body>
<h1>Title</h1>
<p>Some content</p>
<?php
$src_name=basename(__FILE__);
include("/var/www/php_scripts/navblog.php");
?>
</body>
</html>
Replace the path inside of include(""); that is highlighted red above, to wherever you chose to store the script. Make sure that wherever you did choose to store the PHP script, that it's in a location that can be read by the www-data user service account. I recommend storing them somewhere in /var/www.
You’ll also need to configure your web server (Apache or NGINX) to execute PHP from within HTML documents so that this works.
For Apache bros, you'll need to enable the PHP module then do the following:
- Go to '/etc/apache2/mods-enabled' edit 'mime.conf'
- Go to end of file and add the following line: "AddType application/x-httpd-php .html .htm"
- Restart the apache service.
For NGINX bros, I'm not 100% sure since I don't use it, but this is what I found looking around the web. If this doesn't work, then let me know if there's a better method and I'll add it here.
Add the following lines to the nginx configuration file, /etc/nginx.conf:
location ~ \.(php|html|htm)$ {
root html;
fastcgi_pass unix:/var/run/php/php7.4-fpm.sock;
fastcgi_index index.html;
fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name;
include fastcgi_params;
}
Note |
Make sure to adjust the PHP version that is highlighted red in the above code box to the version on your system! |
Shell script index updooter
I’ve also made a shell script that you can re-modify to both create and update the index file(s), which will hold the list of contents you would like to navigate. It makes it easier to keep multiple index files up-to-date this way. You can also add on additional functions to expand the script for different index files.
#!/bin/bash
webroot="cd /var/www/www.cozynet.org/html/"
function update_idx () {
ls -1 | grep -i "$contents" > "/var/www/www.cozynet.org/db/${id}_index.db"
}
function blog_nav_idx () {
eval "$webroot" #Need to move into the web-root of your site to run this
cd blogs/ #This is if you keep your blog posts in a sub-directory.
id='blog' #Set an ID that will be used for the naming of the index file. Make sure this name matches with the file name the PHP script is reading from in the $files variable.
echo "Updating blog index..."
contents='_blog.html' #This variable will be used to search for any files matching the “_blog.html” pattern. Adjust this accordingly for your own site.
update_idx && echo "Completed."
}
blog_nav_idx
Make sure to adjust the above red highlighted to the appropriate location on your web server.
Don’t forget to make the shell script executable! Running it should output a list of files into the “blog_index.db” file, which will then be read by the PHP script to generate the next and previous nav buttons. Any time you create a new blog post, don't forget to updoot that index with the shell script!
Fin
From the visitor perspective, I really like these little page navigation buttons a lot because it makes it easier to just trawl thru whatever is on the site without having to hunt and peck for it. It also improves accessibility!
While static site generators are cool and all, I think applying some PHP scripting in the mix can also greatly improve the maintenance work you would have to put into maintaining a static site. I have a few more scripts and methods that I would like to share in the future, but for now this is all.
Thanks for reading my blog!
Date: 2023-02-19
Back to top!
Comments:
-
Jul 23, 2024
Permalink
Reply
-
Mar 16, 2023
Permalink
Reply
-
Mar 17, 2023
Permalink
Reply
-
Mar 17, 2023
Permalink
Reply